こんにちは、テルプロです!
「Riverpodの使い方がわからない」とお悩みではないでしょうか?
テルプロ
本記事ではそんな悩みを解決していきます!
本記事を読むことで
- Riverpodの使い方をサンプルで理解できる
- コードを公開しているので、自分の環境で確かめることができる
RiverpodでBMI計算アプリを作る
事前準備
パッケージをインストール
今回使用するパッケージは以下の通りです。
dependencies:
flutter:
sdk: flutter
flutter_riverpod: ^1.0.3
font_awesome_flutter: ^10.1.0
dev_dependencies:
flutter_lints: ^1.0.0
flutter_test:
sdk: flutter
プロジェクト構成
リポジトリ構成
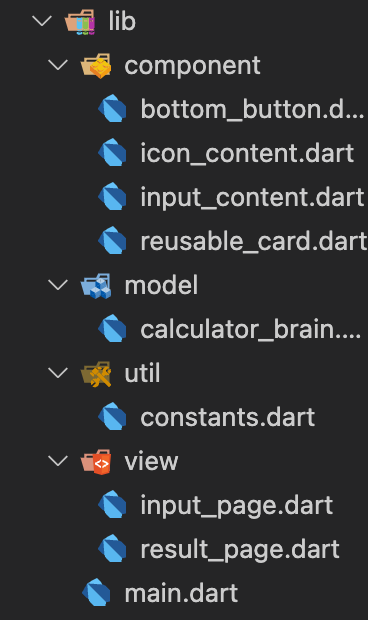
完成イメージ
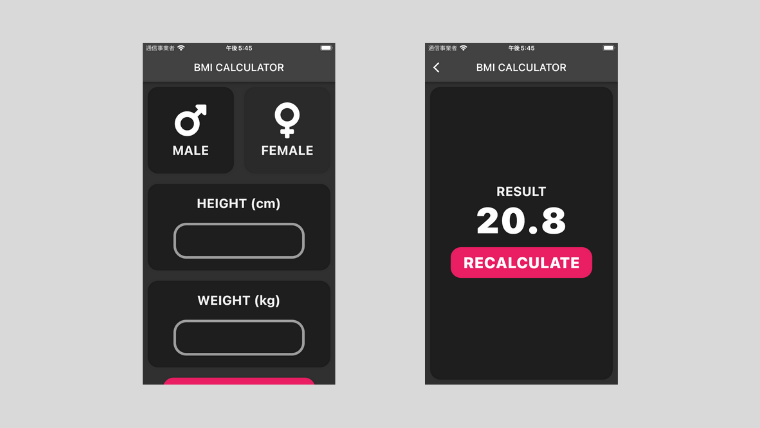
本アプリは2画面構成になります。「高さ」と「身長」を入力し、ボタンを押したら、計算結果が表示されるというシンプルな設計になっています。
ソースコード
main.dart
import 'package:bmi_calculator_app/util/constants.dart';
import 'package:bmi_calculator_app/view/input_page.dart';
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
void main() {
runApp(const ProviderScope(child: MyApp()));
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'BMI CALCULATOR',
theme: ThemeData.dark().copyWith(
inputDecorationTheme: InputDecorationTheme(
border: kTextFieldStyle,
focusedBorder: kTextFieldStyle,
enabledBorder: kTextFieldStyle,
)),
home: HomePage(),
);
}
}
view / input_page.dart
1画面目です。BMIの計算に用いる値を入力するページになります。
import 'dart:math';
import 'package:bmi_calculator_app/component/bottom_button.dart';
import 'package:bmi_calculator_app/component/icon_content.dart';
import 'package:bmi_calculator_app/component/input_content.dart';
import 'package:bmi_calculator_app/component/reusable_card.dart';
import 'package:bmi_calculator_app/util/constants.dart';
import 'package:bmi_calculator_app/model/calculator_brain.dart';
import 'package:bmi_calculator_app/view/result_page.dart';
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
import 'package:font_awesome_flutter/font_awesome_flutter.dart';
class HomePage extends ConsumerWidget {
@override
Widget build(BuildContext context, WidgetRef ref) {
// Providerの監視
final _cardBackground = ref.watch(cardBackgroundProvider.notifier);
final _height = ref.watch(heightProvider.notifier);
final _weight = ref.watch(weightProvider.notifier);
final _bmiValue = ref.watch(bmiValueProvider.notifier);
return Scaffold(
appBar: AppBar(
title: const Text('BMI CALCULATOR'),
),
body: SingleChildScrollView(
child: Container(
height: MediaQuery.of(context).size.height,
child: Column(
children: [
Expanded(
flex: 2,
child: Row(
children: [
Expanded(
child: ReusableCard(
color: ref.watch(cardBackgroundProvider) ==
CardBackground.active
? kActiveCardColor
: kInActiveCardColor,
cardChild: iconContent(FontAwesomeIcons.mars, 'MALE'),
press: () {
_cardBackground.state = CardBackground.active;
},
),
),
Expanded(
child: ReusableCard(
color: ref.watch(cardBackgroundProvider) ==
CardBackground.inActive
? kActiveCardColor
: kInActiveCardColor,
cardChild:
iconContent(FontAwesomeIcons.venus, 'FEMALE'),
press: () {
_cardBackground.state = CardBackground.inActive;
},
),
),
],
),
),
Expanded(
flex: 2,
child: ReusableCard(
color: kActiveCardColor,
cardChild: InputContent(
label: 'HEIGHT (cm)',
inputValue: TextFormField(
keyboardType: TextInputType.number,
style: kInputTextStyle,
textAlignVertical: TextAlignVertical.center,
textAlign: TextAlign.center,
onChanged: (value) {
_height.state = value;
print(value);
},
),
),
),
),
Expanded(
flex: 2,
child: ReusableCard(
color: kActiveCardColor,
cardChild: InputContent(
label: 'WEIGHT (kg)',
inputValue: TextFormField(
keyboardType: TextInputType.number,
style: kInputTextStyle,
textAlignVertical: TextAlignVertical.center,
textAlign: TextAlign.center,
onChanged: (value) {
_weight.state = value;
print(value);
},
),
),
),
),
Container(
margin: EdgeInsets.only(bottom: 20.0),
child: BottomButton(
label: 'CALCULATE',
press: () {
FocusScope.of(context).unfocus();
if (_bmiValue.state == '') {
showDialog(
context: context,
builder: (_) {
return AlertDialog(
content: Text("身長と体重を入力してください。"),
actions: [
TextButton(
child: Text("Cancel"),
onPressed: () => Navigator.pop(context),
),
TextButton(
child: Text("OK"),
onPressed: () => Navigator.pop(context),
),
],
);
},
);
}
_bmiValue.state = CalculatorBrain().getBmiValue(
int.parse(_height.state), int.parse(_weight.state));
print(_bmiValue.state);
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => ResultPage(),
),
);
},
),
),
],
),
),
),
);
}
}
view / result_page.dart
2画面目です。BMIの計算結果を表示するページになります。
import 'package:bmi_calculator_app/component/bottom_button.dart';
import 'package:bmi_calculator_app/component/reusable_card.dart';
import 'package:bmi_calculator_app/util/constants.dart';
import 'package:bmi_calculator_app/model/calculator_brain.dart';
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
class ResultPage extends ConsumerWidget {
@override
Widget build(BuildContext context, WidgetRef ref) {
return Scaffold(
appBar: AppBar(
title: const Text('BMI CALCULATOR'),
),
body: ReusableCard(
color: kActiveCardColor,
cardChild: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'RESULT',
style: kBodyTextStyle,
),
Text(
ref.watch(bmiValueProvider).toString(),
style: kNumberTextStyle,
),
BottomButton(
label: 'RECALCULATE',
press: () {
Navigator.pop(context);
},
),
],
),
),
);
}
}
model / calculator_brain.dart
状態を管理したいものをまとめたファイルです。
カードの背景色とBMIの計算結果を状態として管理しています。
import 'dart:math';
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
/// カードの背景色を管理するための状態管理
enum CardBackground {
active,
inActive,
}
CardBackground? cardBackground;
// Providerの作成
final StateProvider<CardBackground?> cardBackgroundProvider =
StateProvider((ref) => cardBackground);
/// BMIを計算するための状態管理
class CalculatorBrain extends StateNotifier<String> {
CalculatorBrain() : super('');
String getBmiValue(int height, int weight) {
double bmi;
bmi = weight / pow(height / 100, 2);
return bmi.toStringAsFixed(1);
}
}
// Providerの作成
final heightProvider = StateNotifierProvider((ref) => CalculatorBrain());
final weightProvider = StateNotifierProvider((ref) => CalculatorBrain());
final bmiValueProvider = StateNotifierProvider((ref) => CalculatorBrain());
util / constant.dart
UIを構築するにあたり、使い回す値をまとめたファイルです。
import 'package:flutter/material.dart';
const kActiveCardColor = Colors.black38;
const kInActiveCardColor = Colors.black12;
const kButtonColor = Colors.pink;
const kButtonTextStyle = TextStyle(
fontSize: 30.0,
fontWeight: FontWeight.w800,
);
final kTextFieldStyle = OutlineInputBorder(
borderSide: const BorderSide(color: Colors.grey, width: 5.0),
borderRadius: BorderRadius.circular(25.0),
);
const kInputTextStyle = TextStyle(fontSize: 25.0, fontWeight: FontWeight.bold);
const kNumberTextStyle = TextStyle(fontSize: 70.0, fontWeight: FontWeight.w900);
const kBodyTextStyle = TextStyle(
fontSize: 25.0,
fontWeight: FontWeight.w700,
color: Color(0xFFF2F2F2),
);
component / reusable_card.dart
「黒い背景の内容」を再利用可能にするためのファイルです。
import 'package:flutter/material.dart';
class ReusableCard extends StatelessWidget {
const ReusableCard({
Key? key,
required this.cardChild,
required this.color,
this.press,
}) : super(key: key);
final Widget cardChild;
final Color color;
final VoidCallback? press;
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: press,
child: Container(
margin: EdgeInsets.all(10.0),
width: double.infinity,
decoration: BoxDecoration(
color: color,
borderRadius: BorderRadius.circular(20.0),
),
child: cardChild,
),
);
}
}
component / input_content.dart
「TextFieldの内容」を再利用可能にするためのファイルです。
import 'package:bmi_calculator_app/util/constants.dart';
import 'package:flutter/material.dart';
class InputContent extends StatelessWidget {
const InputContent({
Key? key,
required this.label,
required this.inputValue,
}) : super(key: key);
final String label;
final TextFormField inputValue;
@override
Widget build(BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Text(label, style: kBodyTextStyle),
Container(
padding: EdgeInsets.symmetric(horizontal: 50.0),
child: inputValue,
),
],
);
}
}
component / icon_content.dart
「アイコンの内容」を再利用可能にするためのファイルです。
import 'package:bmi_calculator_app/util/constants.dart';
import 'package:flutter/material.dart';
import 'package:font_awesome_flutter/font_awesome_flutter.dart';
class IconContent extends StatelessWidget {
const IconContent({
Key? key,
required this.icon,
required this.label,
}) : super(key: key);
final IconData icon;
final String label;
@override
Widget build(BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Icon(icon, size: 70.0),
const SizedBox(height: 8.0),
Text(label, style: kBodyTextStyle),
],
);
}
}
component / buttom_button.dart
「ボタンの内容」を再利用可能にするためのファイルです。
import 'package:bmi_calculator_app/component/reusable_card.dart';
import 'package:bmi_calculator_app/util/constants.dart';
import 'package:flutter/material.dart';
class BottomButton extends StatelessWidget {
const BottomButton({
Key? key,
required this.label,
required this.press,
}) : super(key: key);
final String label;
final VoidCallback? press;
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: press,
child: Container(
padding: const EdgeInsets.symmetric(horizontal: 30),
height: 80,
child: ReusableCard(
color: kButtonColor,
cardChild: Align(
alignment: Alignment.center,
child: Text(
label,
style: kButtonTextStyle,
textAlign: TextAlign.center,
),
),
),
),
);
}
}
大変お疲れ様でした!以上でアプリは完成です!
今回ご紹介したアプリ全体のソースコードはこちらです。
よろしければ、ご参考にどうぞ。
GitHub:https://github.com/terupro/bmi_calculator_app
まとめ
今回は、Riverpodを用いた「BMI計算アプリの作り方」をご紹介しました。
Riverpodを使うことで状態管理を容易に行うことができます。良ければ上記のコードを参考に、色々と試してみてください。
▼以下では、私の実体験に基づいて「Flutterの効率的な勉強法」の具体的な手順を詳しく解説しています。よろしければ、ご参考にどうぞ!
最後までご覧いただきありがとうございました。ではまた!